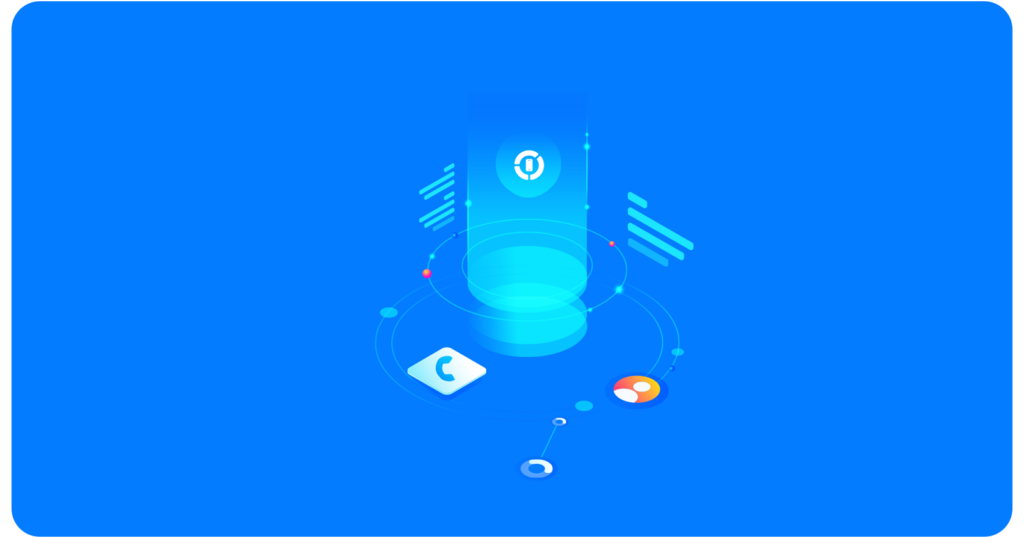
API Overview
Introducing Checky Verify Phone Number API – a powerful tool to determine the validity of phone numbers, retrieve comprehensive carrier information, and discover valid phone numbers within text inputs. Whether you’re building applications in e-commerce, finance, marketing, or other domains, Checky is designed to enhance your services in various ways.
🚀 Features
- Real-time Validation: Determine the validity of a given phone number in real-time.
- Phone Line Identification: Obtain information about the type of phone line connected (e.g., “MOBILE”).
- Carrier Details: Retrieve carrier information for enhanced insights.
- Timezone and Geolocation: Access timezone and geolocation data for a comprehensive understanding.
- Comprehensive Data: Retrieve detailed information about the carrier or service provider.
- Accurate Timezone Data: Access essential timezone information associated with the phone number.
- Enhanced Geolocation: Obtain accurate geolocation information for improved analysis.
- Fraud Prevention: Perfect for identifying and preventing fraudulent activities.
- Text Input Discovery: Discover valid phone numbers within a given text input.
- Data Extraction: Retrieve information about phone type, carrier, timezone, and location.
- Geospatial Analysis: Enhance geospatial analysis with detailed location details.
🏁 Quick Start
Explore the capabilities of Checky and integrate its functionalities to improve your applications, streamline customer interactions, and enhance the security of your services.
- Sign up for an account on RapidAPI and subscribe to the Checky Verify Phone Number.
- Use the provided API key to access the endpoints.
- Start validating phone numbers in your applications or services.
Verify Phone Number
These endpoints determine in realtime if a given phone number is valid and the type of phone line it is connected to. It supports all countries!
Parameters
Parameter | Type | Required | Description |
---|---|---|---|
phone | string | Yes | The phone number to verify. |
country | string | Yes | The two-letter country code of the phone number. eg US, CA, FR etc. |
lang | string | No (Optional) | Preferred language. Default (en). |
/verify
Request Example:
import requests url = "https://checky-verify-phone-number.p.rapidapi.com/verify" querystring = {"phone":"6502530000","country":"US","lang":"en"} headers = { "X-RapidAPI-Key": "SIGN-UP-FOR-KEY", "X-RapidAPI-Host": "checky-verify-phone-number.p.rapidapi.com" } response = requests.get(url, headers=headers, params=querystring) print(response.json())
Request Example:
import requests url = "https://checky-verify-phone-number.p.rapidapi.com/verify/post" payload = { "phone": "6502530000", "country": "US", "lang": "en" } headers = { "content-type": "application/json", "X-RapidAPI-Key": "SIGN-UP-FOR-KEY", "X-RapidAPI-Host": "checky-verify-phone-number.p.rapidapi.com" } response = requests.post(url, json=payload, headers=headers) print(response.json())
JSON Response:
{ "country": "US", "location": "Mountain View, CA", "phone_number": "(650) 253-0000", "phone_type": "FIXED_LINE_OR_MOBILE", "timezone": [ "America/Los_Angeles" ] }
Request Example:
import requests url = "https://checky-verify-phone-number.p.rapidapi.com/verify/batch" payload = { "phone": ["+16502530000", "6502530000"], "country": "US" } headers = { "content-type": "application/json", "X-RapidAPI-Key": "SIGN-UP-FOR-KEY", "X-RapidAPI-Host": "checky-verify-phone-number.p.rapidapi.com" } response = requests.post(url, json=payload, headers=headers) print(response.json())
JSON Response:
{ "results": [ { "country": "US", "location": "Mountain View, CA", "phone_number": "(650) 253-0000", "phone_type": "FIXED_LINE_OR_MOBILE", "timezone": ["America/Los_Angeles"] }, { "country": "US", "location": "Mountain View, CA", "phone_number": "(650) 253-0000", "phone_type": "FIXED_LINE_OR_MOBILE", "timezone": ["America/Los_Angeles"] } ] }
Carrier Info
These endpoints allow you to retrieve information about the carrier or service provider for a given phone number.
Parameters
Parameter | Type | Required | Description |
---|---|---|---|
phone | string | Yes | The phone number for which to retrieve carrier information. |
country | string | Yes | The two-letter country code of the phone number. eg US, CA, FR etc. |
lang | string | No (Optional) | Preferred language. Default (en). |
/carrier
Request Example:
import requests url = "https://checky-verify-phone-number.p.rapidapi.com/carrier" querystring = {"phone":"679009698","country":"CM"} headers = { "X-RapidAPI-Key": "SIGN-UP-FOR-KEY", "X-RapidAPI-Host": "checky-verify-phone-number.p.rapidapi.com" } response = requests.get(url, headers=headers, params=querystring) print(response.json())
Request Example:
import requests url = "https://checky-verify-phone-number.p.rapidapi.com/carrier/post" payload = { "phone": "679009698", "country": "CM", "lang": "fr" } headers = { "content-type": "application/json", "X-RapidAPI-Key": "SIGN-UP-FOR-KEY", "X-RapidAPI-Host": "checky-verify-phone-number.p.rapidapi.com" } response = requests.post(url, json=payload, headers=headers) print(response.json())
JSON Response:
{ "carrier": "MTN Cameroon", "country_code": "CM", "location": "Cameroun", "phone_number": [ true, "MOBILE", "6 79 00 96 98" ] }
Request Example:
import requests url = "https://checky-verify-phone-number.p.rapidapi.com/carrier/batch" payload = { "phone": ["679009698", "620063770"], "country": "CM" } headers = { "content-type": "application/json", "X-RapidAPI-Key": "SIGN-UP-FOR-KEY", "X-RapidAPI-Host": "checky-verify-phone-number.p.rapidapi.com" } response = requests.post(url, json=payload, headers=headers) print(response.json())
JSON Response:
{ "results": [ { "carrier": "MTN Cameroon", "country_code": "CM", "location": "Cameroon", "phone_number": [ true, "MOBILE", "6 79 00 96 98" ] }, { "carrier": "Camtel", "country_code": "CM", "location": "Cameroon", "phone_number": [ true, "MOBILE", "6 20 06 37 70" ] } ] }
Extract Phone Numbers
This endpoint allows you to find valid phone numbers in a given text input. It validates the phone numbers and provide information about them, such as phone type, carrier, timezone, and geolocation.
Parameters
Parameter | Type | Required | Description |
---|---|---|---|
text | string | Yes | The input text in which you want to find valid phone numbers. |
country | string | Yes | The two-letter country code of the phone number. eg US, CA, FR etc. |
lang | string | No (Optional) | Preferred language. Default (en). |
/finder
Request Example:
import requests url = "https://checky-verify-phone-number.p.rapidapi.com/finder" payload = { "text": "Call me at 510-748-8230 if it's before 9:30, or on 703-4800500 after 10am.", "country": "US" } headers = { "content-type": "application/json", "X-RapidAPI-Key": "SIGN-UP-FOR-KEY", "X-RapidAPI-Host": "checky-verify-phone-number.p.rapidapi.com" } response = requests.post(url, json=payload, headers=headers) print(response.json())
JSON Response:
{ "phone_numbers": [ { "carrier": "", "country": "US", "location": "Alameda, CA", "phone_number": "(510) 748-8230", "phone_type": "FIXED_LINE_OR_MOBILE", "timezone": "America/Los_Angeles" }, { "carrier": "", "country": "US", "location": "Virginia", "phone_number": "(703) 480-0500", "phone_type": "FIXED_LINE_OR_MOBILE", "timezone": "America/New_York" } ] }
🎉 Benefits of Using Checky API
- Enhanced Data Quality: Ensure accuracy in your database by validating phone numbers.
- Fraud Prevention: Identify and prevent fraudulent activities through phone number verification.
- Geospatial Analysis: Improve geospatial analysis and location-based services with accurate geolocation data.
- Customer Trust: Build trust with customers by validating their phone numbers and ensuring secure communication.
- Versatility: Suitable for a wide range of use cases, including e-commerce, finance, marketing, and more.
- Sending emails to valid and engaged recipients improves sender reputation and increases email deliverability rates, ultimately boosting campaign success.
🔧 Issues
If you encounter any issues or have suggestions for improvement, please open an issue on GitHub. We appreciate your feedback!